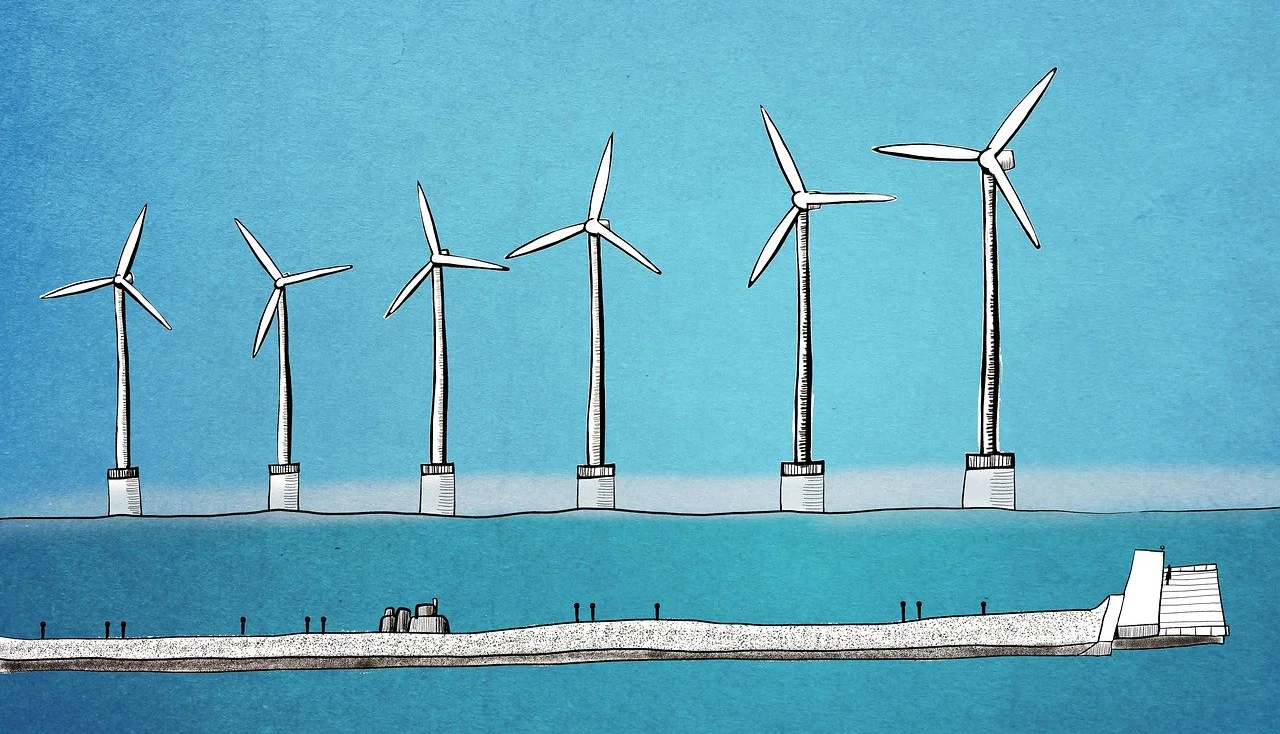
对原生AJAX 的简单封装:wink:
我们先看一下JQuery的AJAX的使用。
jQuery.ajax(url,[settings])
参数:
- url:一个用来包含发送请求的URL字符串。
- data [Object,String]类型:发送到服务器的数据。将自动转换为请求字符串格式。GET 请求中将附加在 URL 后。查看 processData 选项说明以禁止此自动转换。必须为 Key/Value 格式。如果为数组,jQuery 将自动为不同值对应同一个名称。如 {foo:[“bar1”, “bar2”]} 转换为 “&foo=bar1&foo=bar2”。
- type [String]类型:(默认: “GET”) 请求方式 (“POST” 或 “GET”), 默认为 “GET”。注意:其它 HTTP 请求方法,如 PUT 和 DELETE 也可以使用,但仅部分浏览器支持。
- success 当请求之后调用。传入返回后的数据,以及包含成功代码的字符串。
- error 在请求出错时调用。传入XMLHttpRequest对象,描述错误类型的字符串以及一个异常对象(如果有的话)
JQuery AJAX 的简单使用
1 2 3 4 5 6 7 8 9 10 11
| $.ajax({ type: "get", url: "ajax_get.php", data: "username=John&userpwd=Boston", success: function(xrh) { alert(xrh.responseText); }, error: function() { alert("请求错误") } });
|
对原生AJAX的封装:
对元素我们需要注意的有以下几点
- 对IE5 IE6的兼容
- AJAX在ie中的缓存问题
- url中的中文进行转码 地址栏中不能出现中文
- 将传入的对象转化为字符串函数
- 判断外界是否传入超时
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
|
function datastr(data) { var res = []; data.t = new Date().getTime(); for (var key in data) { res.push(encodeURIComponent(key) + "=" + encodeURIComponent(data[key])); } return res.join("&"); }
function ajax(option) {
var str = datastr(option.data); var xhr, timer; if (window.XMLHttpRequest) { xhr = new XMLHttpRequest(); } else { xhr = new ActiveXObject("Microsoft.XMLHTTP"); } if (option.type.toLowerCase() === "get") { xhr.open(option.type, option.url + "?" + str, true); xhr.send(); } else { xhr.open(option.type, option.url, true); xhr.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); xhr.send(str); }
xhr.onreadystatechange = function() { if (xhr.readyState === 4) { clearInterval(timer); if (xhr.status >= 200 && xhr.status <= 300 || xhr.status == 304) { option.success(xhr); option.option.complete();; } else { option.error(xhr); option.complete(); } } }
if (option.timeout) { timer = setInterval(function() { xhr.abort(); clearInterval(timer); }, option.timeout) }
}
|
对封装的AJAX的简单使用:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| btn.onclick = function() { ajax({ type: "get", url: "1.txt", timeout: 3000, data: { "username": "wzg", "userpwd": "123" }, success: function(xhr) { let x = xhr.responseText; y = JSON.parse(x); console.log(y.name); console.log(y.age); }, error: function(xhr) { alert("请求失败!!!"); } }); }
|