JS 手机号/身份证/银行账号/邮箱/QQ/电话 掩码处理
手机号掩码:
1 | //手机号掩码 |
身份证号掩码:
1 | //身份证号掩码: |
银行账号掩码:
1 | //银行账号掩码 |
邮箱掩码:
1 | //邮箱掩码 |
QQ掩码:
1 | //qq掩码 |
电话掩码:
1 | //电话掩码 |
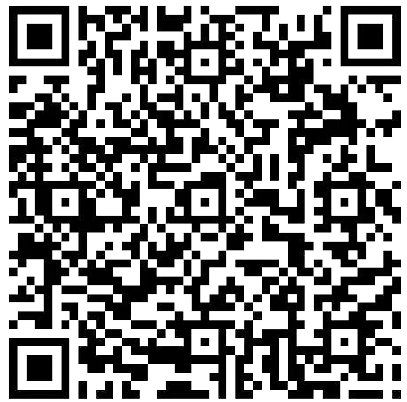
Invitation
aqing
962555446
created:15/04/2021
Welcome to Candyhome
Use this card to join the candyhome and participate in a pleasant discussion together .
Welcome to aqing's candyhome,wish you a nice day .
评论