vue子组件的created和mounted中获取不到props中接收到的值
父子组件通信:
父组件中通过v-bind绑定传送,子组件通过props接收
例如:
父组件:
1 | <template> |
子组件:
1 | export default { |
这种情况下,子组件的 methods 中想要取到props中的值,直接使用 this.chartData 即可
但是有写情况下,你的 chartData 里面的值并不是固定的,而是动态获取的,这种情况下,你会发现 methods 中是取不到你的 chartData 的,或者取到的一直是默认值。
比如下面这种情况:
1 | <script> |
此时子组件的methods中使用 this.chartData 会发现是不存在的(因为为空了)
这情况我是使用watch处理:
解决方法如下:
使用 watch :
1 | export default { |
监听 chartData 的值,当它由空转变时就会触发,这时候就能取到了,拿到值后要做的处理方法也需要在 watch 里面执行。
//总结
出现这种情况的原因, 因为父组件中的要就要传递的 props 属性 是通过 发生ajax请求回来的, 请求的这个过程是需要时间的,但是子组件的渲染要快于ajax请求过程,所以此时 created 、 mounted 这样的只会执行一次的生命周期钩子,已经执行了,但是 props 还没有流进来(子组件),所以只能拿到默认值。
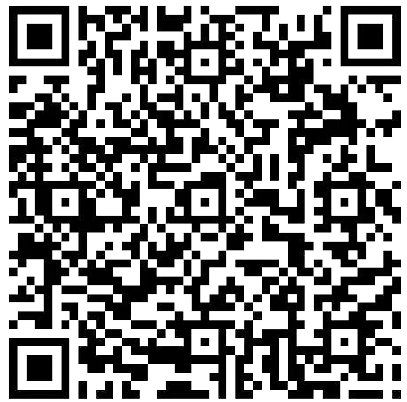
Invitation
aqing
962555446
created:15/04/2021
Welcome to Candyhome
Use this card to join the candyhome and participate in a pleasant discussion together .
Welcome to aqing's candyhome,wish you a nice day .
评论