React父子组件之间传值
父组件给子组件传值
1. class类组件,父组件在子组件上写入属性,子组件通过this.props.属性名获取值
1. 函数式组件,父组件在子组件上写入属性,子组件需要把props作为组件参数才能使用props
子组件给父组件传值
- 通过传递回调函数的方式,父组件给子组件传一个回调函数(可带参数),子组件通过this.props.回调函数名,通过调用回调函数,传入参数来传值
一.父组件向子组件传值
在父组件中引入子组件,将父组件的state
值放到子组件标签中传递,在子组件中用this.props.传值名称,接收
父组件代码:
1 | import React, { Component } from 'react' |
子组件代码:
1 | import React, { Component } from 'react' |
还可以设置默认值 首先引入 prop-types 代码如下:
1 | import PropTypes from 'prop-types' |
Goods是组件名 title是默认值名称 Title是默认值
也可以校验传值的类型 代码如下:
1 | import PropTypes from 'prop-types' |
PropTypes.string是检查是否为字符串
其他的是:
检查值是否为数组
PropTypes.array
检查值是否为布尔类型
PropTypes.bool
检查值是否为函数
PropTypes.func
检查值是否为数字
PropTypes.number
检查值是否为对象
PropTypes.object
检查值是否为Symbol类型
PropTypes.symbol
二、子组件传递父组件
通过this.props.名称(值)传给父组件,父组件在子组件标签中定义方法接收修改渲染的this.state值;代码如下:
子组件代码:
1 | import React, { Component } from 'react' |
父组件代码:
1 | import Goods from '../Good/Goods' |
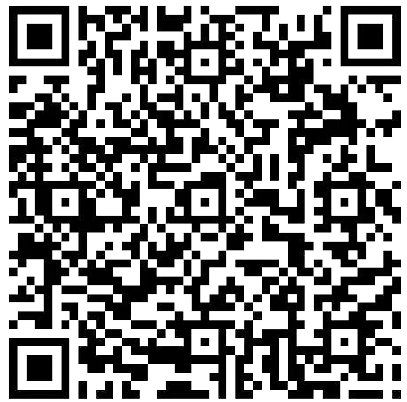
Invitation
aqing
962555446
created:15/04/2021
Welcome to Candyhome
Use this card to join the candyhome and participate in a pleasant discussion together .
Welcome to aqing's candyhome,wish you a nice day .
评论