父组件需要向子组件传递一个函数,然后,子组件通过props获取函数并附上参数,最后,父组件通过函数拿到子组件传递的值。
父组件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| import React, { useState } from 'react'; import Child from './component/child'; import './index.less'; const Home: React.FC = () => { const [parentCount, setParentCountt] = useState<number>(0); const getChildCount = (val: number) => { setParentCountt(val); }; return ( <div className="home-wrap"> <p>我是父组件</p> <p>子组件传过来的数字:{parentCount}</p> <Child getCount={getChildCount} /> </div> ); }; export default Home;
|
子组件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| import React, { useState } from 'react'; type selfProps = { getCount: Function; }; const Child: React.FC<selfProps> = (props) => { const { getCount } = props; const [count, setCount] = useState<number>(0); const addCount = (val: number) => { setCount(val); getCount(val); }; return ( <div className="child-wrap"> <p>子组件</p> <p>数字:{count}</p> <button onClick={() => addCount(count + 1)}>数字递增</button> </div> ); }; export default Child;
|
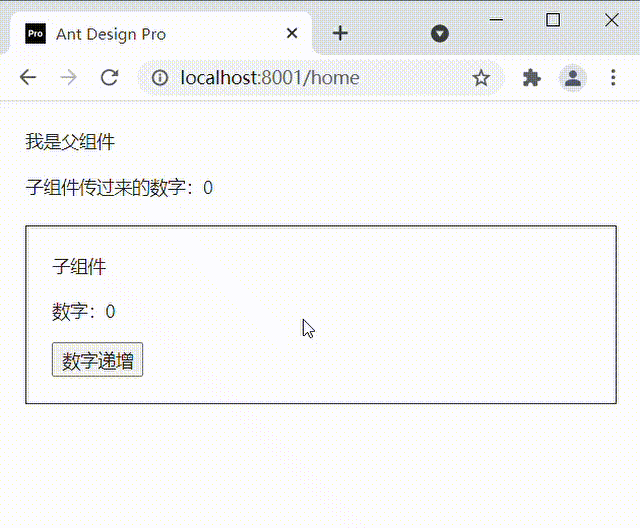