react函数式组件传值之父传子
我们在引入子组件时,子组件的名字一定要大写,否则会报错(这是个babel编译机制问题)。那么,父组件在引入子组件后,如何传值呢?首先,父组件要将传递的参数写到子组件标签上,然后,子组件通过props接收父组件传过来的所有参数。
组件Home向子组件Child传递count值,子组件通过props拿到此值并渲染出来。
父组件:
1 | import React, { useState } from 'react'; |
子组件:
1 | import React from 'react'; |
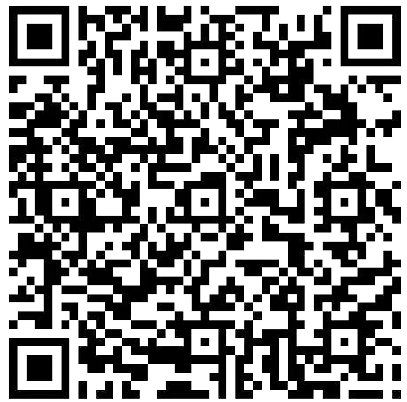
Invitation
aqing
962555446
created:15/04/2021
Welcome to Candyhome
Use this card to join the candyhome and participate in a pleasant discussion together .
Welcome to aqing's candyhome,wish you a nice day .
评论